How To Play An Animation In Java
Animation
concluding modified July 20, 2020
In this part of the Java 2D games tutorial, we will work with blitheness.
Animation
Animation is a rapid display of sequence of images which creates an illusion of movement. We will animate a star on our Board. We will implement the movement in three basic ways. Nosotros will apply a Swing timer, a standard utility timer, and a thread.
Animation is a complex subject in game programming. Java games are expected to run on multiple operating systems with different hardware specifications. Threads requite the most accurate timing solutions. However, for our unproblematic 2D games, other two options tin can be an option likewise.
Swing timer
In the first example we will use a Swing timer to create animation. This is the easiest just too the to the lowest degree constructive way of animative objects in Java games.
SwingTimerEx.java
package com.zetcode; import java.awt.EventQueue; import javax.swing.JFrame; public class SwingTimerEx extends JFrame { public SwingTimerEx() { initUI(); } private void initUI() { add(new Board()); setResizable(fake); pack(); setTitle("Star"); setLocationRelativeTo(null); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } public static void primary(String[] args) { EventQueue.invokeLater(() -> { SwingTimerEx ex = new SwingTimerEx(); ex.setVisible(true); }); } }
This is the main class for the code example.
setResizable(false); pack();
The setResizable()
sets whether the frame tin can exist resized. The pack()
method causes this window to be sized to fit the preferred size and layouts of its children. Note that the order in which these two methods are called is important. (The setResizable()
changes the insets of the frame on some platforms; calling this method after the pack()
method might pb to incorrect results—the star would not go precisely into the right-bottom edge of the window.)
Lath.java
package com.zetcode; import java.awt.Colour; import java.awt.Dimension; import coffee.awt.Graphics; import java.awt.Paradigm; import java.awt.Toolkit; import java.awt.outcome.ActionEvent; import java.awt.event.ActionListener; import javax.swing.ImageIcon; import javax.swing.JPanel; import javax.swing.Timer; public course Board extends JPanel implements ActionListener { private final int B_WIDTH = 350; private last int B_HEIGHT = 350; private final int INITIAL_X = -40; individual final int INITIAL_Y = -40; private last int DELAY = 25; private Epitome star; private Timer timer; private int x, y; public Board() { initBoard(); } private void loadImage() { ImageIcon ii = new ImageIcon("src/resources/star.png"); star = two.getImage(); } individual void initBoard() { setBackground(Colour.Blackness); setPreferredSize(new Dimension(B_WIDTH, B_HEIGHT)); loadImage(); x = INITIAL_X; y = INITIAL_Y; timer = new Timer(Delay, this); timer.commencement(); } @Override public void paintComponent(Graphics g) { super.paintComponent(thousand); drawStar(thou); } private void drawStar(Graphics g) { g.drawImage(star, ten, y, this); Toolkit.getDefaultToolkit().sync(); } @Override public void actionPerformed(ActionEvent e) { x += 1; y += ane; if (y > B_HEIGHT) { y = INITIAL_Y; x = INITIAL_X; } repaint(); } }
In the Lath
class we movement a star that from the upper-left corner to the right-bottom corner.
individual final int B_WIDTH = 350; individual last int B_HEIGHT = 350; private final int INITIAL_X = -twoscore; private final int INITIAL_Y = -xl; private final int Filibuster = 25;
5 constants are defined. The commencement two constants are the lath width and height. The third and fourth are the initial coordinates of the star. The terminal one determines the speed of the animation.
private void loadImage() { ImageIcon ii = new ImageIcon("src/resources/star.png"); star = 2.getImage(); }
In the loadImage()
method we create an instance of the ImageIcon
class. The prototype is located in the project directory. The getImage()
method volition return the the Paradigm
object from this class. This object will be drawn on the lath.
timer = new Timer(DELAY, this); timer.start();
Here we create a Swing Timer
class and call its start()
method. Every DELAY
ms the timer will telephone call the actionPerformed()
method. In social club to use the actionPerformed()
method, we must implement the ActionListener
interface.
@Override public void paintComponent(Graphics 1000) { super.paintComponent(yard); drawStar(thou); }
Custom painting is done in the paintComponent()
method. Note that we also call the paintComponent()
method of its parent. The actual painting is delegated to the drawStar() method.
private void drawStar(Graphics g) { g.drawImage(star, x, y, this); Toolkit.getDefaultToolkit().sync(); }
In the drawStar() method, we depict the image on the window with the usage of the drawImage()
method. The Toolkit.getDefaultToolkit().sync()
synchronises the painting on systems that buffer graphics events. Without this line, the animation might not be shine on Linux.
@Override public void actionPerformed(ActionEvent e) { x += 1; y += 1; if (y > B_HEIGHT) { y = INITIAL_Y; 10 = INITIAL_X; } repaint(); }
The actionPerformed()
method is repeatedly called by the timer. Inside the method, we increment the ten and y values of the star object. Then we call the repaint()
method which will crusade the paintComponent()
to be called. This style we regularly repaint the Board
thus making the animation.
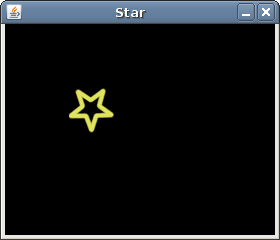
Utility timer
This is very similar to the previous manner. We use the java.util.Timer
instead of the javax.Swing.Timer
. For Java Swing games this manner is more accurate.
UtilityTimerEx.java
package com.zetcode; import java.awt.EventQueue; import javax.swing.JFrame; public class UtilityTimerEx extends JFrame { public UtilityTimerEx() { initUI(); } private void initUI() { add(new Board()); setResizable(imitation); pack(); setTitle("Star"); setLocationRelativeTo(null); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } public static void main(String[] args) { EventQueue.invokeLater(() -> { JFrame ex = new UtilityTimerEx(); ex.setVisible(true); }); } }
This is the primary class.
Lath.coffee
bundle com.zetcode; import coffee.awt.Color; import java.awt.Dimension; import java.awt.Graphics; import java.awt.Image; import java.awt.Toolkit; import coffee.util.Timer; import java.util.TimerTask; import javax.swing.ImageIcon; import javax.swing.JPanel; public class Board extends JPanel { private final int B_WIDTH = 350; private last int B_HEIGHT = 350; private final int INITIAL_X = -40; private last int INITIAL_Y = -40; individual concluding int INITIAL_DELAY = 100; private final int PERIOD_INTERVAL = 25; private Image star; individual Timer timer; private int x, y; public Board() { initBoard(); } private void loadImage() { ImageIcon ii = new ImageIcon("src/resources/star.png"); star = ii.getImage(); } individual void initBoard() { setBackground(Colour.BLACK); setPreferredSize(new Dimension(B_WIDTH, B_HEIGHT)); loadImage(); x = INITIAL_X; y = INITIAL_Y; timer = new Timer(); timer.scheduleAtFixedRate(new ScheduleTask(), INITIAL_DELAY, PERIOD_INTERVAL); } @Override public void paintComponent(Graphics yard) { super.paintComponent(g); drawStar(g); } private void drawStar(Graphics grand) { g.drawImage(star, 10, y, this); Toolkit.getDefaultToolkit().sync(); } individual class ScheduleTask extends TimerTask { @Override public void run() { x += 1; y += 1; if (y > B_HEIGHT) { y = INITIAL_Y; x = INITIAL_X; } repaint(); } } }
In this case, the timer will regularly call the run()
method of the ScheduleTask
class.
timer = new Timer(); timer.scheduleAtFixedRate(new ScheduleTask(), INITIAL_DELAY, PERIOD_INTERVAL);
Here nosotros create a timer and schedule a job with a specific interval. There is an initial filibuster.
@Override public void run() { ... }
Each 10 ms the timer volition call this run()
method.
Thread
Animating objects using a thread is the most effective and authentic way of blitheness.
ThreadAnimationEx.java
packet com.zetcode; import coffee.awt.EventQueue; import javax.swing.JFrame; public class ThreadAnimationEx extends JFrame { public ThreadAnimationEx() { initUI(); } private void initUI() { add(new Board()); setResizable(false); pack(); setTitle("Star"); setLocationRelativeTo(nix); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } public static void main(Cord[] args) { EventQueue.invokeLater(() -> { JFrame ex = new ThreadAnimationEx(); ex.setVisible(true); }); } }
This is the main class.
Board.coffee
package com.zetcode; import java.awt.Color; import coffee.awt.Dimension; import java.awt.Graphics; import java.awt.Image; import coffee.awt.Toolkit; import javax.swing.ImageIcon; import javax.swing.JOptionPane; import javax.swing.JPanel; public grade Board extends JPanel implements Runnable { private final int B_WIDTH = 350; private concluding int B_HEIGHT = 350; private concluding int INITIAL_X = -40; private final int INITIAL_Y = -xl; private terminal int DELAY = 25; private Image star; private Thread animator; private int x, y; public Board() { initBoard(); } private void loadImage() { ImageIcon 2 = new ImageIcon("src/resource/star.png"); star = 2.getImage(); } private void initBoard() { setBackground(Color.BLACK); setPreferredSize(new Dimension(B_WIDTH, B_HEIGHT)); loadImage(); 10 = INITIAL_X; y = INITIAL_Y; } @Override public void addNotify() { super.addNotify(); animator = new Thread(this); animator.commencement(); } @Override public void paintComponent(Graphics g) { super.paintComponent(grand); drawStar(thou); } private void drawStar(Graphics thou) { grand.drawImage(star, x, y, this); Toolkit.getDefaultToolkit().sync(); } private void cycle() { x += 1; y += 1; if (y > B_HEIGHT) { y = INITIAL_Y; x = INITIAL_X; } } @Override public void run() { long beforeTime, timeDiff, sleep; beforeTime = System.currentTimeMillis(); while (true) { cycle(); repaint(); timeDiff = System.currentTimeMillis() - beforeTime; slumber = DELAY - timeDiff; if (sleep < 0) { sleep = 2; } attempt { Thread.sleep(sleep); } take hold of (InterruptedException due east) { String msg = Cord.format("Thread interrupted: %s", east.getMessage()); JOptionPane.showMessageDialog(this, msg, "Error", JOptionPane.ERROR_MESSAGE); } beforeTime = System.currentTimeMillis(); } } }
In the previous examples, nosotros executed a task at specific intervals. In this example, the animation will have place inside a thread. The run()
method is called merely once. This is why why nosotros have a while loop in the method. From this method, we call the cycle()
and the repaint()
methods.
@Override public void addNotify() { super.addNotify(); animator = new Thread(this); animator.start(); }
The addNotify()
method is called later our JPanel
has been added to the JFrame
component. This method is often used for various initialisation tasks.
We desire our game run smoothly, at constant speed. Therefore we compute the system fourth dimension.
timeDiff = System.currentTimeMillis() - beforeTime; slumber = DELAY - timeDiff;
The cycle()
and the repaint()
methods might take different time at various while cycles. We calculate the time both methods run and decrease it from the Filibuster
constant. This mode nosotros want to ensure that each while cycle runs at constant time. In our case, it is Filibuster
ms each wheel.
This part of the Java 2d games tutorial covered animation.
Source: https://zetcode.com/javagames/animation/
Posted by: proctortweat1979.blogspot.com
0 Response to "How To Play An Animation In Java"
Post a Comment